To give you an idea how easy it is to use
Direct I/O we would like to show you step-by-step how to integrate
Direct I/O with your own application.
Let's imagine you have to write an application named MYAPP.EXE to control a special
hardware with I/O ports in the range 310-31Fh.
- Enter the resources needed:
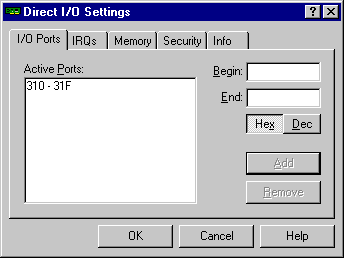
- Give your application the right to access these resources:

- Write your application:
Note: During debugging you may have to add your development application to the Security
section (see 2.) too.
Some debuggers - for example VB - execute your code under their control.
And that's all folks! As I said... Easy.
// part of the really cool application MYAPP
HANDLE hDevice;
hDevice = CreateFile(
"\\\\.\\DirectIo0", // pointer to name of the file
GENERIC_READ | GENERIC_WRITE, // access (read-write) mode
FILE_SHARE_READ | FILE_SHARE_WRITE, // share mode
NULL, // pointer to security attributes
OPEN_EXISTING, // how to create
0, // file attributes
NULL); // handle to file with attributes to copy
if (hDevice == INVALID_HANDLE_VALUE) // did we succeed?
{
// possibly call GetLastError() to get a hint what failed
// and terminate (it is useless to continue if we cant connect to Direct I/O)
}
// some more processing...
while (_inp(0x310) & 0x01) // loop while not ready
;
_outpw(0x312, 0x1234); // write a secret word
|
' We have to call the Win32 API (see Direct I/O online help)
' Declare the neccessary functions and constants
Private Declare Function CreateFile Lib "KERNEL32" Alias "CreateFileA" ( _
ByVal lpFileName As String, _
ByVal dwDesiredAccess As Long, _
ByVal dwShareMode As Long, _
ByVal lpSecurityAttributes As Long, _
ByVal dwCreationDisposition As Long, _
ByVal dwFlagsAndAttributes As Long, _
ByVal hTemplateFile As Long) As Long
Private Const GENERIC_READ As Long = &H80000000
Private Const GENERIC_WRITE As Long = &H40000000
Private Const FILE_SHARE_READ As Long = 1
Private Const FILE_SHARE_WRITE As Long = 2
Private Const OPEN_EXISTING As Long = 3
Private Const INVALID_HANDLE_VALUE As Long = -1
' Unfortunately (?!?) VB doesn't provide functions for hardware access.
' So we have to take a 3rd party supplement (there are many freeware libs around,
' search the web for Win95io.zip or inpout32.zip).
' The following code is taken from Win95io:
Private Declare Sub vbOut Lib "WIN95IO.DLL" ( _
ByVal nPort As Integer, _
ByVal nData As Integer)
Private Declare Sub vbOutw Lib "WIN95IO.DLL" ( _
ByVal nPort As Integer, _
ByVal nData As Integer)
Private Declare Function vbInp Lib "WIN95IO.DLL" ( _
ByVal nPort As Integer) As Integer
Private Declare Function vbInpw Lib "WIN95IO.DLL" ( _
ByVal nPort As Integer) As Integer
Private Sub Form_Load()
Dim hDevice As Long
hDevice = CreateFile("\\.\DirectIo0", GENERIC_READ Or GENERIC_WRITE, _
FILE_SHARE_READ Or FILE_SHARE_WRITE, 0&, OPEN_EXISTING, 0&, 0&)
If hDevice = INVALID_HANDLE_VALUE Then
' ask what went wrong
MsgBox "Unable to open driver (error code = " & Err.LastDllError & _
"). Aborting..."
' terminate (it is useless to continue if we cant connect to Direct I/O)
End
End If
End Sub
Private Sub Read_Click()
Value = vbInp(Port)
End Sub
Private Sub Write_Click()
vbOut Port, Value
End Sub
|
Download the support DLL for the sample above:
Note: Ignore the warnings about
Windows NT. You have Direct I/O :-)
This sample has been provided by Claudio
Klingler
unit directio;
interface
uses
Windows, Messages, SysUtils, Classes, Graphics, Controls, Forms, Dialogs, WinTypes;
type
TForm1 = class(TForm)
procedure FormCreate(Sender: TObject);
private
{ Private declarations }
public
{ Public declarations }
end;
var
Form1: TForm1;
implementation
{$R *.DFM}
function PortIn(IOAddr : WORD) : WORD;
begin
asm
mov dx,IOAddr
in ax,dx
mov result,ax
end;
end;
procedure PortOut(IOAddr : WORD; Data : WORD);
begin
asm
mov dx,IOAddr
mov ax,Data
out dx,ax
end;
end;
procedure TForm1.FormCreate(Sender: TObject);
var hDevice : HWND;
begin
hDevice := CreateFile(
'\\.\DirectIo0', // pointer to name of the file
GENERIC_READ or GENERIC_WRITE, // access (read-write) mode
FILE_SHARE_READ or FILE_SHARE_WRITE, // share mode
NIL, // pointer to security attributes
OPEN_EXISTING, // how to create
0, // file attributes
0); // handle to file with attributes to copy
if (hDevice = INVALID_HANDLE_VALUE) then // did we succeed?
begin
Application.MessageBox('Error', 'error', MB_OK);
// possibly call GetLastError() to get a hint what failed
// and terminate (it is useless to continue if we cant connect to Direct I/O)
end;
// some more processing...
while (PortIn($0310) and $01 = $01) do
begin
// Loop
end;
PortOut($0312, $01234); // write a secret word
end;
end.
|
|